Model Context Protocol (MCP) finally gives AI models a way to access the business data needed to make them really useful at work. CData MCP Servers have the depth and performance to make sure AI has access to all of the answers.
Try them now for free →Create a Data Access Object for Workday Data using JDBI
A brief overview of creating a SQL Object API for Workday data in JDBI.
JDBI is a SQL convenience library for Java that exposes two different style APIs, a fluent style and a SQL object style. The CData JDBC Driver for Workday integrates connectivity to live Workday data in Java applications. By pairing these technologies, you gain simple, programmatic access to Workday data. This article walks through building a basic Data Access Object (DAO) and the accompanying code to read and write Workday data.
About Workday Data Integration
CData provides the easiest way to access and integrate live data from Workday. Customers use CData connectivity to:
- Access the tables and datasets you create in Prism Analytics Data Catalog, working with the native Workday data hub without compromising the fidelity of your Workday system.
- Access Workday Reports-as-a-Service to surface data from departmental datasets not available from Prism and datasets larger than Prism allows.
- Access base data objects with WQL, REST, or SOAP, getting more granular, detailed access but with the potential need for Workday admins or IT to help craft queries.
Users frequently integrate Workday with analytics tools such as Tableau, Power BI, and Excel, and leverage our tools to replicate Workday data to databases or data warehouses. Access is secured at the user level, based on the authenticated user's identity and role.
For more information on configuring Workday to work with CData, refer to our Knowledge Base articles: Comprehensive Workday Connectivity through Workday WQL and Reports-as-a-Service & Workday + CData: Connection & Integration Best Practices.
Getting Started
Create a DAO for the Workday Workers Entity
The interface below declares the desired behavior for the SQL object to create a single method for each SQL statement to be implemented.
public interface MyWorkersDAO {
//insert new data into Workday
@SqlUpdate("INSERT INTO Workers (Legal_Name_Last_Name, Legal_Name_Last_Name) values (:legal_Name_Last_Name, :legal_Name_Last_Name)")
void insert(@Bind("legal_Name_Last_Name") String legal_Name_Last_Name, @Bind("legal_Name_Last_Name") String legal_Name_Last_Name);
//request specific data from Workday (String type is used for simplicity)
@SqlQuery("SELECT Legal_Name_Last_Name FROM Workers WHERE Legal_Name_Last_Name = :legal_Name_Last_Name")
String findLegal_Name_Last_NameByLegal_Name_Last_Name(@Bind("legal_Name_Last_Name") String legal_Name_Last_Name);
/*
* close with no args is used to close the connection
*/
void close();
}
Open a Connection to Workday
Collect the necessary connection properties and construct the appropriate JDBC URL for connecting to Workday.
To connect to Workday, users need to find the Tenant and BaseURL and then select their API type.
Obtaining the BaseURL and Tenant
To obtain the BaseURL and Tenant properties, log into Workday and search for "View API Clients." On this screen, you'll find the Workday REST API Endpoint, a URL that includes both the BaseURL and Tenant.
The format of the REST API Endpoint is: https://domain.com/subdirectories/mycompany, where:
- https://domain.com/subdirectories/ is the BaseURL.
- mycompany (the portion of the url after the very last slash) is the Tenant.
Using ConnectionType to Select the API
The value you use for the ConnectionType property determines which Workday API you use. See our Community Article for more information on Workday connectivity options and best practices.
API | ConnectionType Value |
---|---|
WQL | WQL |
Reports as a Service | Reports |
REST | REST |
SOAP | SOAP |
Authentication
Your method of authentication depends on which API you are using.
- WQL, Reports as a Service, REST: Use OAuth authentication.
- SOAP: Use Basic or OAuth authentication.
See the Help documentation for more information on configuring OAuth with Workday.
Built-in Connection String Designer
For assistance in constructing the JDBC URL, use the connection string designer built into the Workday JDBC Driver. Either double-click the JAR file or execute the jar file from the command-line.
java -jar cdata.jdbc.workday.jar
Fill in the connection properties and copy the connection string to the clipboard.
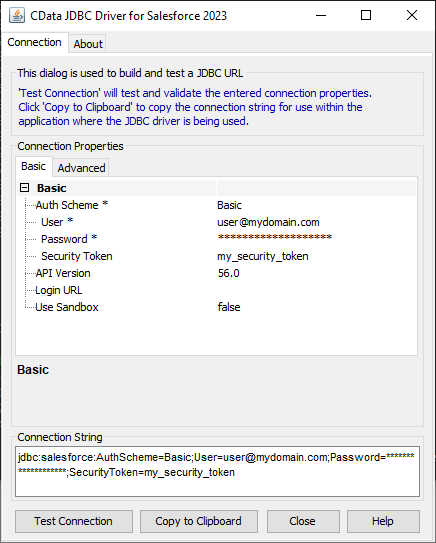
A connection string for Workday will typically look like the following:
jdbc:workday:User=myuser;Password=mypassword;Tenant=mycompany_gm1;BaseURL=https://wd3-impl-services1.workday.com;ConnectionType=WQL;InitiateOAuth=GETANDREFRESH
Use the configured JDBC URL to obtain an instance of the DAO interface. The particular method shown below will open a handle bound to the instance, so the instance needs to be closed explicitly to release the handle and the bound JDBC connection.
DBI dbi = new DBI("jdbc:workday:User=myuser;Password=mypassword;Tenant=mycompany_gm1;BaseURL=https://wd3-impl-services1.workday.com;ConnectionType=WQL;InitiateOAuth=GETANDREFRESH");
MyWorkersDAO dao = dbi.open(MyWorkersDAO.class);
//do stuff with the DAO
dao.close();
Read Workday Data
With the connection open to Workday, simply call the previously defined method to retrieve data from the Workers entity in Workday.
//disply the result of our 'find' method
String legal_Name_Last_Name = dao.findLegal_Name_Last_NameByLegal_Name_Last_Name("Morgan");
System.out.println(legal_Name_Last_Name);
Write Workday Data
It is also simple to write data to Workday, using the previously defined method.
//add a new entry to the Workers entity
dao.insert(newLegal_Name_Last_Name, newLegal_Name_Last_Name);
Since the JDBI library is able to work with JDBC connections, you can easily produce a SQL Object API for Workday by integrating with the CData JDBC Driver for Workday. Download a free trial and work with live Workday data in custom Java applications today.